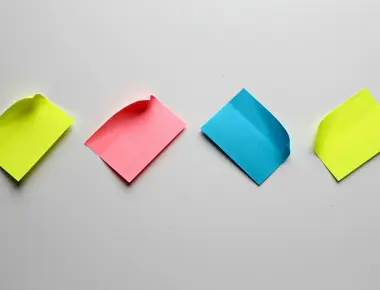
This is a very simple C program to reverse all the characters in a given string. As for example considering the string “hello world”, the program should output “dlrow olleh”. The string reversal problem is a common embedded software interview question asked for both entry level and middle level firmware engineering positions.
#include <stdio.h> void strRev(char *str) { char temp; char *begin = str; char *end = str; if (!str || !(*str)) goto error; // Go to the end of the string while (*(++end)) ; // Discard the null character end--; while (begin != end) { // Swap temp = *end; *end = *begin; *begin = temp; // Move the pointers begin++; end--; } error: return; } int main(void) { char str[] = "hello world"; puts(str); strRev(str); puts(str); return 0; }
This simple C program is to reverse a string. Reversing a string is a classic interview question which is asked commonly. In this given example, the function strRev() reverses the characters present in the character array.
The character pointers begin and end are assigned with the address of the received string str.
char *begin = str; char *end = str;
The lines,
if (!str || !(*str)) goto error;
checks for a null pointer or for a null value and jumps to error: label.
A character pointer end is moved to the last character (excluding the null character) in the received string in the following lines,
// Go to the end of the string while (*(++end)) ; // Discard the null character end--;
Finally the begin and end pointers’ dereferenced characters are swapped until all characters are swapped.
while (begin != end) { // Swap temp = *end; *end = *begin; *begin = temp; // Move the pointers begin++; end--; }
Now the string characters are reversed and the new string is printed from main().
Quick Links
Legal Stuff