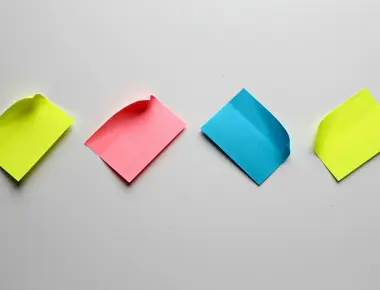
This is a common embedded software interview bitwise operation question. The magic numbers ‘5’ and ‘10’ are used arbitrarily to make the understanding of the problem easier. The idea of this program is to set 5 bits of a word starting from the bit position 10. Code walk-through and algorithm is explained below.
#include <stdio.h> void print_32bit_binary(int x) { int i; for (i = 0; i < 32; i++) { ((1 << 31) & (x << i)) ? putchar('1') : putchar('0'); } putchar('\n'); } int main(void) { int x = 10; print_32bit_binary(x); // set 5 bits starting from position 10 in x int temp; temp = ~(~0 << 5) << (10 + 1 - 5); print_32bit_binary(x | temp); return 0; }
This simple C program demonstrates the use of bitwise operators to set 5 bits starting from position 10. This example also implements a helper function print_32bit_binary() to print values in binary representation.
The given number is printed in its binary form by calling the function print_32bit_binary() from main().
The function print_32bit_binary() prints the int number in its binary form by looping through the int bit-width and outputs a character 1 or 0 after testing the corresponding bit position,
void print_32bit_binary(int x) { int i; for (i = 0; i < 32; i++) { ((1 << 31) & (x << i)) ? putchar('1') : putchar('0'); } putchar('\n'); }
Coming to the actual problem of setting the 5 bits starting from position 10 is by creating a bitmask at line,
temp = ~(~0 << 5) << (10 + 1 - 5);
and ORing the bitmask with the given number at line,
print_32bit_binary(x | temp);
From the statement, temp = ~(~0 << 5) << (10 + 1 - 5); the code section ~(~0 << 5) creates a value which has only the last 5 bits set to 1. Then the code section (10 + 1 - 5) will point to the 10th bit position (1 is added since the bit position starts from 0) for bitwise operation and 5 is subtracted since 5 bits needs to be set.
Quick Links
Legal Stuff