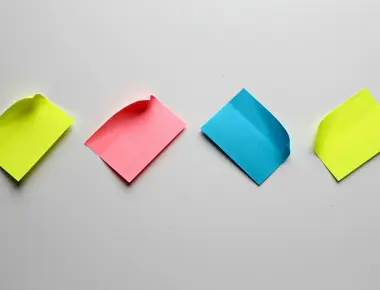
This is a very interesting clock angle problem where the C program described in this article calculates the angle between the hour-hand and the minute-hand of an analog clock. In this example program if the user enters the hour and minute, then it should output the angle between the hour-hand and the minute-hand.
Example:
Input: hour = 10 and minute = 10
Output: 115 degree
The hour hand of a 12-hour analog clock turns 3600 in (12 x 60) minutes; in other words 0.50 per minute. Similarly, the minute hand would take 60 minutes to turn a complete 3600 (which is 60 per minute). The angle between the hour hand and minute hand is obtained by the difference between these angles.
#include <stdio.h> #include <stdlib.h> int main() { unsigned int hour; unsigned int minute; unsigned int hAngle; unsigned int mAngle; unsigned int angle; printf("Enter hour (12h format): "); scanf("%u", &hour); printf("Enter minute: "); scanf("%u", &minute); if (hour > 12u || minute > 60u) { printf("Invalid input!\n"); } else { if (hour == 12u) { hour = 0; } if (minute == 60u) { minute = 0; hour += 1u; } hAngle = 0.5f * ((hour * 60u) + minute); // Hour hand angle with respect to 12:00 mAngle = 6u * minute; // Minute hand angle with respect to 12:00 angle = abs(hAngle - mAngle); if (angle > (360u - angle)) { angle = 360u - angle; // Getting the smaller angle value } printf("Angle between hour hand and minute hand is %d\n", angle); } return 0; }
The code is simple and self explanatory. Angle between the hour hand and the minute hand is calculated at the below lines,
hAngle = 0.5f * ((hour * 60u) + minute); // Hour hand angle with respect to 12:00 mAngle = 6u * minute; // Minute hand angle with respect to 12:00
abs() library function is used to get the absolute value of the angle difference in angle = abs(hAngle - mAngle);
The below code section selects the smaller angle value from the two possible angle values,
if (angle > (360u - angle)) { angle = 360u - angle; // Getting the smaller angle value }
Quick Links
Legal Stuff