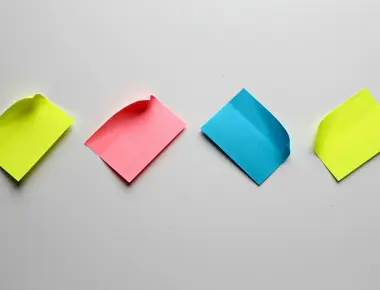
The unary operator ‘sizeof’ is a very handy tool in C as well as in C++. It gives the storage size of a data-type/variable. This article describes an example C program showing the custom implementation of the ‘sizeof’ operator using macros.
#include <stdio.h>#define MYSIZEOF(X) (char *)(&(X) + 1) - (char *)(&(X))int main(void){int x;printf("%d\n", MYSIZEOF(x));return 0;}
This example is an implementation of a custom sizeof() operator in C. The sizeof() operator functionality is implemented here using the macro function MYSIZEOF().
The code section, (char )(&(X) + 1)* adds 1 to the pointer according to the pointer type and the resultant address is type-casted to a character pointer. Correlating with the example, when the address of the integer variable x is added with 1 then the address is advanced by 4 bytes (considering 4 bytes for int). In the right hand side of the - operator, the address of the variable is type-casted to a character pointer.
Now the expression (char *)(&(X) + 1) - (char *)(&(X)) gives the size of the variable.
Quick Links
Legal Stuff